Watcom C Library Reference : ecvt, _ellipse, _ellipse_w, _ellipse_wxy, _enable, _endthread, eof
Watcom C Reference/D - E - F 2021. 6. 23. 17:41
Watcom C Library Reference : ecvt, _ellipse, _ellipse_w, _ellipse_wxy, _enable, _endthread, eof
ecvt
Synopsis : #include <stdlib.h>
char *ecvt ( double value, int ndigits, int *dec, int *sign );
Description : The ecvt function converts the floating-point number value into a character string. The parameter ndigits specifies the number of significant digits desired. The converted number will be rounded to ndigits of precision.
The character string will contain only digits and is terminated by a null character The integer pointed to by dec will be filled in with a value indicating the position of the decimal point relative to the start of the string of digits. A zero or negative value indicates that the decimal point lies to the left of the first digit. The integer pointed to by sign will contain 0 if the number is positive, and non-zero if the number is negative.
Returns : The ecvt function returns a pointer to a static buffer containing the converted string of digits. Note: ecvt and fcvt both use the same static buffer.
See Also : fcvt, govt, printf
Example :
#include <stdio.h>
#include <stdlib.h>
void main( )
{
char *str;
int dec, sign;
str = ecvt ( 123.456789, 6, &dec, &sign );
printf( "str=%s, dec=%d, sign=%d\n", str, dec, sign );
}
produces the following :
str=123457, dec=3, sign=0
Classification : WATCOM
Systems : All
_ellipse, _ellipse_w, _ellipse_wxy
Synopsis : include <graph.h>
short _FAR _ellipse( short fill, short x1, short y1, short x2, short y2 );
short _FAR _ellipse_w( short fill, double x1, double y1, double x2, double y2 );
short _FAR _ellipse_wxy ( short fill,
struct _wxycoord _FAR *pi,
struct _wxycoord _FAR *p2 );
Description : The _ellipse functions draw ellipses. The _ellipse function uses the view coordinate system. The _ellipse_w and _ellipse_wxy functions use the window coordinate system.
The center of the ellipse is the center of the rectangle established by the points (x1, y1) and (x2, y2).
The argument fill determines whether the ellipse is filled in or has only its outline drawn. The argument can have one of two values:
Value | Meaning |
_GFILLINTERIOR | fill the interior by writing pixels with the current plot action using the current color and the current fill mask |
_GBORDER | leave the interior unchanged; draw the outline of the figure with the current plot action using the current color and line style |
When the coordinates (x1, y1) and (x2, y2) establish a line or a point (this happens when one or more of the x-coordinates or y-coordinates are equal), nothing is drawn.
Returns : The _ellipse functions return a non-zero value when the ellipse was successfully drawn; otherwise, zero is returned.
See Also : _arc, _rectangle, _setcolor, _setfillmask, _setlinestyle. _setplotaction
Example :
#include <conio.h>
#include <graph.h>
void main( )
{
_setvideomode ( _VRES16COLOR );
_ellipse( _GBORDER, 120, 90, 520, 390 );
getch();
_setvideomode ( _DEFAULTMODE );
}
produces the following :
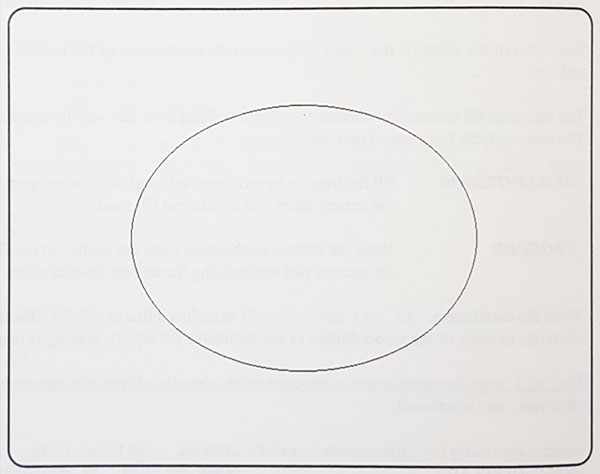
Classification : PC Graphics
Systems : _ellipse - DOS, QNX
_ellipse_w - DOS, QNX
_ellipse_wxy - DOS, QNX
_enable
Synopsis : #include <i86.h>
void _enable( void );
Description : The _enable function causes interrupts to become enabled. The _enable function would be used in conjunction with the _disable function to make sure that a sequence of instructions are executed without any intervening interrupts occurring.
Returns : The _enable function returns no value.
See Also : _disable
Example :
#include <stdio.h>
#include <stdlib.h>
#include <i86.h>
struct list_entry {
struct list_entry *next;
int data;
};
struct list_entry *ListHead = NULL;
struct list_entry *ListTail = NULL;
void insert ( struct list_entry *new_entry)
{
/* insert new_entry at end of linked list */
new_entry->next = NULL;
_disable( ); /* disable interrupts */
if( ListTail == NULL ) {
ListHead = new_entry;
} else {
ListTail->next = new_entry;
}
ListTail = new_entry;
_enable(); /* enable interrupts now */
}
void main( )
{
struct list_entry *p;
int i;
for( i = 1; i <= 10; i++ ) {
p = ( struct list_entry *) malloc ( sizeof( struct list_entry ) );
if ( p == NULL ) break;
p->data = i;
insert ( p );
}
}
Classification : Intel
Systems : All
_endthread
Synopsis : #include <process.h>
void __far _endthread (void);
Description : The _endthread function uses the OS/2 function DosExit to end the current thread of execution.
Returns : The _endthread function does not return any value.
See Also : _beginthread
Example :
#include <stdio.h>
#include <stdlib.h>
#include <process.h>
#define STACK_SIZE 4096
#if defined ( __386__)
#define FAR
#else
#define FAR __far
#endif
void FAR child( void FAR *parm )
{
char * FAR *argv = (char * FAR *) parm;
int i;
for( i = 0; argv[i]; i++ ) {
printf( "argv[%d] = %s\n", i, argv[i] );
}
_endthread ( );
}
void main( )
{
char *stack;
char *args[3];
int tid;
args[0] = "child";
args[1] = "parm";
args[2] = NULL;
stack = (char *) malloc ( STACK_SIZE );
tid = _beginthread ( child, stack, STACK_SIZE, args );
}
Classification : OS/2
Systems : OS/2 1.x(MT), OS/2 1.x(DL), OS/2 2.x, NT
eof
Synopsis : #include <io.h>
int eof( int handle );
Description : The eof function determines, at the operating system level, if the end of the file has been reached for the file whose file handle is given by handle. Because the current file position is set following an input operation, the eof function may be called to detect the end of the file before an input operation beyond the end of the file is attempted.
Returns : The eof function returns 1 if the current file position is at the end of the file. O if the current file position is not at the end. A return value of -1 indicates an error and in this case errno is set to indicate the error.
Errors : When an error has occurred, errno contains a value indicating the type of error that has been detected.
Constant | Meaning |
EBADF | The handle argument is not a valid file handle. |
See Also : read
Example :
#include <stdio.h>
#include <fcntl.h>
#include <io.h>
void main( )
{
int handle, len;
char buffer[100];
handle = open( "file", O_RDONLY );
if( handle != -1 ) {
while( ! eof ( handle ) ) {
len = read ( handle, buffer, sizeof (buffer) - 1);
buffer[ len ] = '\0';
printf( "%s", buffer );
}
close ( handle );
}
}
Classification : WATCOM
Systems : All
This manual describes the WATCOM C library for DOS, Windows, and OS/2, It includes the Standard C Library (as defined in the ANSI C Standard).
WATCOM C Language Reference manual describes the ANSI C Programming language and extensions to it which are supported by WATCOM C/C++ (32bit)