Watcom C Library : _getwindowcoord, gmtime Functions, _grow_handles, _grstatus, _grtext, _grtext_w
Watcom C Reference/G - H - I 2021. 6. 30. 13:10
Watcom C Library Reference : _getwindowcoord, gmtime Functions, _grow_handles, _grstatus, _grtext, _grtext_w
_getwindowcoord
Synopsis : #include <graph.h>
struct _wxycoord _FAR _getwindowcoord( short x, short y );
Description : The _getwindowcoord function returns the window coordinates of the position with view coordinates (x, y). Window coordinates are defined by the _setwindow function.
Returns : The _getwindowcoord function returns the window coordinates, as a _wxycoord structure, of the given point.
See Also : _setwindow, _getviewcoord
Example :
#include <conio.h>
#include <graph.h>
void main( )
{
struct xycoord centre;
struct _wxycoord pos1, pos2;
/* draw a box 50 pixels square in the middle of the screen */
_setvideomode( _MAXRESMODE );
centre = _getviewcoord_w ( 0.5, 0.5 );
pos1 = _getwindowcoord( centre.xcoord - 25, centre.ycoord - 25 );
pos2 = _getwindowcoord( centre.xcoord + 25, centre.ycoord + 25 );
_rectangle_wxy ( _GBORDER, &pos1, &pos2 );
getch( );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
gmtime Functions
Synopsis : #include <time.h>
struct tm * gmtime( const time_t *timer ) ;
struct tm *_gmtime( const time_t *timer, struct tm *tmbuf );
struct tm {
int tm_sec; /* seconds after the minute -- [0,61] */
int tm_min; /* minutes after the hour -- [0,59] */
int tm_hour; /* hours after midnight -- [0,23] */
int tm_mday; /* day of the month -- [1,31] */
int tm_mon; /* months since January -- [0,11] */
int tm_year; /* years since 1900 */
int tm_wday; /* days since Sunday -- [0,6] */
int tm_yday; /* days since January 1 -- [0,365] */
int tm_isdst; /* Daylight Savings Time flag */
};
Description : The gmtime functions convert the calendar time pointed to by timer into a broken-down time, expressed as Coordinated Universal Time (UTC) (formerly known as Greenwich Mean Time (GMT)).
The function _gmtime places the converted time in the tm structure pointed to by tmbuf, and the function gmtime places the converted time in a static structure that is re-used each time gmtime is called.
The time set on the computer with the DOS time command and the DOS date command reflects the local time. The environment variable TZ is used to establish the time zone to which this local time applies. See the section The TZ Environment Variable for a discussion of how to set the time zone.
Returns : The gmtime functions return a pointer to a structure containing the broken-down time.
See Also : asctime, clock, ctime, difftime, localtime, mktime, strftime, time, tzset
Example :
#include <stdio.h>
#include <time.h>
void main( )
{
time_t time_of_day;
auto char buf[26] ;
auto struct tm tmbuf;
time_of_day = time( NULL );
_gmtime ( &time_of_day, &tmbuf );
printf( "It is now : %.24s GMT\n", _asctime ( &tmbuf, buf ) );
}
produces the following :
It is now : Fri Dec 25 15:58:27 1999 GMT
Classification : gmtime is ANSI, _gmtime is not ANSI
Systems : gmtime - All
_gmtime - All
_grow_handles
Synopsis : #include <stdio.h>
int _grow_handles( int new_count );
Description : The _grow_handles function increases the number of POSIX level files that are allowed to be open at one time. The parameter new_count is the new requested number of files that are allowed to be opened. The return value is the number that is allowed to be opened after the call. This may be less than, equal to, or greater than the number requested. If the number is less than, an error has occurred and the errno variable should be consulted for the reason. If the number returned is greater than or equal to the number requested, the call was successful.
Note that even if _grow_handles returns successfully, you still might not be able to open the requested number of files due to some system limit (e.g. FILES= in the CONFIG.SYS file under DOS) or because some file handles are already in use (stdin, stdout, stderr, etc.).
Returns : The _grow_handles function returns the new number of file handles which may be open simultaneously.
Errors : When an error has occurred, errno contains a value indicating the type of error that has been detected.
See Also : fdopen, fopen, open, tmpfile
Example :
#include <stdio.h>
FILE *fp[50];
void main( )
{
int hndl_count;
int i;
hndl_count = _NFILES;
if( hndl_count < 50 ) {
hndl_count = _grow_handles( 50 );
}
for ( i = 0; i < hndl_count; i++ ) {
fp[i] = tmpfile( );
if( fp[i] == NULL ) break;
printf( "File %d successfully opened\n", i );
}
printf( "%d files were successfully opened\n", i );
}
Classification : WATCOM
Systems : All
_grstatus
Synopsis : #include <graph.h>
short _FAR _grstatus( void );
Description : The _grstatus function returns the status of the most recently called graphics library function. The function can be called after any graphics function to determine if any errors or warnings occurred. The function returns 0 if the previous function was successful. Values less than 0 indicate an error occurred; values greater than 0 indicate a warning condition.
The following values can be returned:
Constant | Value | Explanation |
_GROK | 0 | no error |
_GRERROR | -1 | graphics error |
_GRMODENOTSUPPORTED | -2 | video mode not supported |
_GRNOTINPROPERMODE | -3 | function n/a in this mode |
_GRINVALIDPARAMETER | -4 | invalid parameter (s) |
_GRINSUFFICIENTMEMORY | -5 | out of memory |
_GRFONTFILENOTFOUND | -6 | can't open font file |
_GRINVALIDFONTFILE | -7 | font file has invalid format |
_GRNOOUTPUT | 1 | nothing was done |
_GRCLIPPED | 2 | output clipped |
Returns : The _grstatus function returns the status of the most recently called graphics library function.
Example :
#include <conio.h>
#include <graph.h>
#include <stdlib.h>
void main( )
{
int x, y;
_setvideomode( _VRES16COLOR );
while( _grstatus ( ) == _GROK ) {
x = rand( ) % 700;
y = rand( ) % 500;
_setpixel( x, y );
}
getch( );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
_grtext, _grtext_w
Synopsis : #include <graph.h>
short _FAR _grtext( short x, short y, char _FAR *text ) ;
short _FAR _grtext_w( double x, double y, char _FAR *text );
Description : The _grtext functions display a character string. The -grtext function uses the view coordinate system. The _grtext_w function uses the window coordinate system.
The character string text is displayed at the point (x, y). The string must be terminated by a null character ('\0'). The text is displayed in the current color using the current text settings.
The graphics library can display text in three different ways.
1. The _outtext and _outmem functions can be used in any video mode. However, this variety of text can be displayed in only one size. | |
2. The _grtext function displays text as a sequence of line segments, and can be drawn in different sizes, with different orientations and alignments. | |
3. The _outgtext function displays text in the currently selected font. Both bit-mapped and vector fonts are supported; the size and type of text depends on the fonts that are available. |
Returns : The _grtext functions return a non-zero value when the text was successfully drawn: otherwise, zero is returned.
See Also : _outtext, _outmem, _outgtext, _setcharsize, _settextalign, _settextpath, _settextorient, _setcharspacing
Example :
#include <conio.h>
#include <graph.h>
void main( )
{
_setvideomode( _VRES16COLOR );
_grtext ( 200, 100, "WATCOM" );
_grtext ( 200, 200, "Graphics" );
getch( );
_setvideomode( _DEFAULTMODE );
}
produces the following :
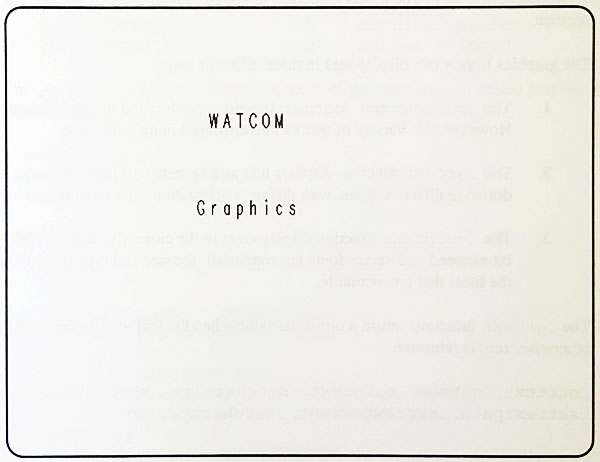
Classification : PC Graphics
Systems : _grtext - DOS, QNX
_grtext_w - DOS, QNX
This manual describes the WATCOM C library for DOS, Windows, and OS/2, It includes the Standard C Library (as defined in the ANSI C Standard).
WATCOM C Language Reference manual describes the ANSI C Programming language and extensions to it which are supported by WATCOM C/C++ (32bit)