Watcom C Library : ispunct, isspace, isupper, isxdigit, itoa
Watcom C Reference/G - H - I 2021. 7. 6. 15:49
Watcom C Library Reference : ispunct, isspace, isupper, isxdigit, itoa
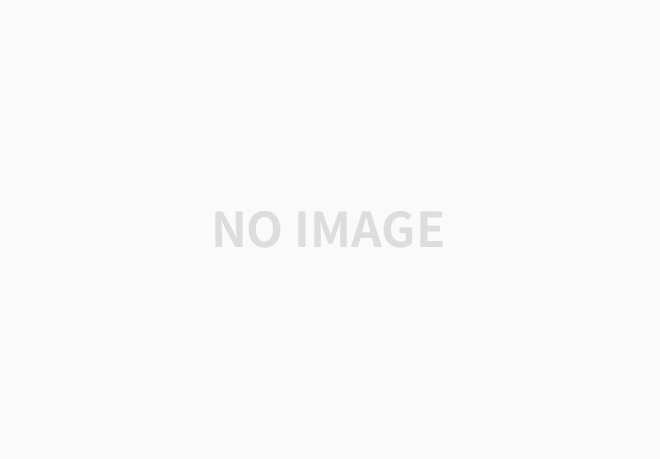
ispunct
Synopsis : #include <ctype.h>
int ispunct( int c );
Description : The ispunct function tests for any punctuation character such as a comma (,) or a period (.)
Returns : The ispunct function returns a non-zero value when the argument is a punctuation character; otherwise, zero is returned.
See Also : isalnum, isalpha, iscntrl, isdigit, isgraph, islower, isprint, isspace, isupper, isxdigit, tolower, toupper
Example :
#include <stdio.h>
#include <ctype.h>
char chars [ ] = {
'A',
'!',
'.',
',',
':',
';'
};
#define SIZE sizeof( chars ) / sizeof( char )
void main( )
{
int i;
for( i = 0; i < SIZE; i++ ) {
printf( "Char %c is %s a punctuation character\n",
chars[i], ( ispunct( chars[i] ) ) ? "" : "not " );
}
}
produces the following :
Char A is not a punctuation character
Char ! is a punctuation character
Char , is a punctuation character
Char , is a punctuation character
Char : is a punctuati on character
Char ; is a punctuation character
Classification : ANSI
Systems : All
isspace
Synopsis : #include <ctype.h>
int isspace( int c );
Description : The isspace function tests for the following white-space characters:
Constant | Character |
' ' | space |
'\f' | form feed |
'\n' | new-line or linefeed |
'\r' | carriage return |
'\t' | horizontal tab |
'\v' | vertical tab |
Returns : The isspace function returns a non-zero character when the argument is one of the indicated white-space characters; otherwise, zero is returned.
See Also : isalnum, isalpha, iscntrl, isdigit, isgraph, islower, isprint, ispunct, isupper, isxdigit, tolower, toupper
Example :
#include <stdio.h>
#include <ctype.h>
char chars[ ] = {
'A',
0x09,
' ',
0x7d
};
#define SIZE sizeof( chars ) / sizeof( char )
void main( )
{
int i;
for( i = 0; i < SIZE; i++ ) {
printf( "Char %c is %s a space character\n",
chars(i), ( isspace ( chars[i] ) ) ? "" : "not" );
}
}
produces the following :
Char A is not a space character
Char is a space character
Char is a space character
Char } is not a space character
Classification : ANSI
Systems : All
isupper
Synopsis : #include <ctype.h>
int isupper( int c );
Description : The isupper function tests for any uppercase letter 'A' through 'Z'.
Returns : The isupper returns a non-zero value when the argument is an uppercase letter; otherwise, zero is returned.
See Also : isalnum, isalpha, iscntrl, isdigit, isgraph, islower, isprint, ispunct, isspace, isxdigit, tolower, toupper
Example :
#include <stdio.h>
#include <ctype.h>
char chars [ ] = {
'A',
'a',
'z',
'Z'
};
#define SIZE sizeof( chars ) / sizeof( char )
void main( )
{
int i;
for ( i = 0; i < SIZE; i++ ) {
printf( "Char %c is %s an uppercase character\n",
chars[i], ( isupper( chars[i] ) ) ?" : "not " );
}
}
produces the following :
Char A is an uppercase character
Char a is not an uppercase character
Char z is not an uppercase character
Char Z is an uppercase character
Classification : ANSI
Systems : All
isxdigit
Synopsis : #include <ctype.h>
int isxdigit( int c );
Description : The isxdigit function tests for any hexadecimal-digit character. These characters are the digits ('0' through '9') and the letters ('a' through 'f') and ('A' through 'F').
Returns : The isxdigit function returns a non-zero value when the argument is a hexadecimal digit; otherwise, zero is returned.
See Also : isalnum, isalpha, iscntrl, isdigit, isgraph, islower, isprint, ispunct, isspace, isupper, tolower, toupper
Example :
#include <stdio.h>
#include <ctype.h>
char chars[ ] = {
'A',
'5',
'$'
};
#define SIZE sizeof( chars ) / sizeof( char )
void main( )
{
int i;
for ( i = 0; i < SIZE; i++ ) {
printf( "Char %c is %s a hexadecimal digit character\n",
chars[i], ( isxdigit( chars[i] ) ) ? "" : "not " );
}
}
produces the following :
Char A is a hexadecimal digit character
Char 5 is a hexadecimal digit character
Char $ is not a hexadecimal digit character
Classification : ANSI
Systems : All
itoa
Synopsis : #include <stdlib.h>
char *itoa( int value, char *buffer, int radix );
Description : The itoa function converts the binary integer value into the equivalent string in base radix notation storing the result in the character array pointed to by buffer. A null character is appended to the result. The size of buffer must be at least (8 * sizeof(int) + 1) bytes when converting values in base 2. That makes the size 17 bytes on 16-bit machines, and 33 bytes on 32-bit machines. If the value of radix is 10 and value is negative, then a minus sign is prepended to the result.
Returns : The itoa function returns the pointer to the result.
See Also : atoi, strtol, utoa
Example :
#include <stdio.h>
#include <stdlib.h>
void main( )
{
char buffer [20];
int base;
for( base = 2; base <= 16; base = base + 2 ) {
printf( "%2d %s\n", base, itoa( 12765, buffer, base ) );
}
}
produces the following :
2 11000111011101
4 3013131
6 135033
8 30735
10 12765
12 7479
14 491b
16 31dd
Classification : WATCOM
Systems : All
This manual describes the WATCOM C library for DOS, Windows, and OS/2, It includes the Standard C Library (as defined in the ANSI C Standard).
WATCOM C Language Reference manual describes the ANSI C Programming language and extensions to it which are supported by WATCOM C/C++ (32bit)