Watcom C Library : _gettextcolor, _gettextcursor, _gettextextent, _gettextposition, _gettextsettings
Watcom C Reference/G - H - I 2021. 6. 29. 18:02
Watcom C Library Reference : _gettextcolor, _gettextcursor, _gettextextent, _gettextposition, _gettextsettings
_gettextcolor
Synopsis : #include <graph.h>
short _FAR _gettextcolor( void );
Description : The _gettextcolor function returns the pixel value of the current text color. This is the color used for displaying text with the _outtext and _outmem functions. The default text color value is set to 7 whenever a new video mode is selected.
Returns : The _gettextcolor function returns the pixel value of the current text color.
See Also : _settextcolor, _setcolor, _outtext, _outmem
Example :
#include <conio.h>
#include <graph.h>
main( )
{
int old_col;
long old_bk;
_setvideomode( _TEXTC80 );
old_col = _gettextcolor( );
old_bk = _getbkcolor( );
_settextcolor( 7 );
_setbkcolor( _BLUE );
_outtext( " WATCOM \nGraphics" );
_settextcolor( old_col );
_setbkcolor( old_bk );
getch( );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
_gettextcursor
Synopsis : #include <graph.h>
short _FAR _gettextcursor( void );
Description : The _gettextcursor function returns the current cursor attribute, or shape. The cursor shape is set with the _settextcursor function. See the _settextcursor function for a description of the value returned by the _gettextcursor function.
Returns : The _gettextcursor function returns the current cursor shape when successful; otherwise, (-1) is returned.
See Also : _settextcursor, _displaycursor
Example :
#include <conio.h>
#include <graph.h>
main( )
{
int old_shape;
old_shape = _gettextcursor( );
_settextcursor( 0x0007 );
_outtext ( "\nBlock cursor" );
getch( );
_settextcursor( 0x0407 );
_outtext( "\nHalf height cursor" );
getch( );
_settextcursor( 0x2000 );
_outtext( "\nNo cursor" );
getch( );
_settextcursor( old_shape );
}
Classification : PC Graphics
Systems : DOS, QNX
_gettextextent
Synopsis : #include <graph.h>
void _FAR _gettextextent( short x, short y,
char _FAR *text,
struct xycoord _FAR *concat,
struct xycoord _FAR *extent ) ;
Description : The _gettextextent function simulates the effect of using the _grtext function to display the text string text at the position (x, y), using the current text settings. The concatenation point is returned in the argument concat. The text extent parallelogram is returned in the array extent.
The concatenation point is the position to use to output text after the given string. The text extent parallelogram outlines the area where the text string would be displayed. The four points are returned in counter-clockwise order, starting at the upper-left corner.
Returns : The _gettextextent function does not return a value.
See Also : _grtext, _gettextsettings
Example :
#include <conio.h>
#include <graph.h>
main( )
{
struct xycoord concat;
struct xycoord extent[4];
_setvideomode( _VRES16COLOR );
_grtext ( 100, 100, "hot" );
_gettextextent( 100, 100, "hot", Concatenation Functions, &extent );
_polygon( _GBORDER, 4, &extent );
_grtext( concat.xcoord, concat.ycoord, "dog" );
getch( );
_setvideomode( _DEFAULTMODE );
}
produces the following :
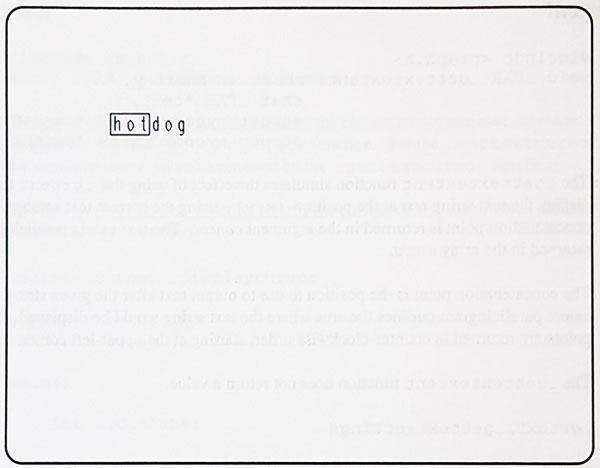
Classification : PC Graphics
Systems : DOS, QNX
_gettextposition
Synopsis : #include <graph.h>
struct rccoord _FAR _gettextposition( void );
Description : The _gettextposition function returns the current output position for text. This position is in terms of characters, not pixels.
The current position defaults to the top left corner of the screen, (1, 1), when a new video mode is selected. It is changed by successful calls to the _outtext, _outmem, _settextposition and _settextwindow functions.
Note that the output position for graphics output differs from that for text output. The output position for graphics output can be set by use of the _moveto function.
Returns : The _gettextposition function returns, as an rccoord structure, the current output position for text.
See Also : _outtext, _outmem, _settextposition, _settextwindow, _moveto
Example :
#include <conio.h>
#include <graph.h>
main( )
{
struct rccoord old_pos;
_setvideomode( _TEXTC80 );
old_pos = _gettextposition( );
_settextposition( 10, 40 );
_outtext ( "WATCOM Graphics" );
_settextposition( old_pos.row, old_pos.col );
getch( );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
_gettextsettings
Synopsis : #include <graph.h>
struct textsettings _FAR * _FAR _gettextsettings( struct textsettings _FAR *settings );
Description : The _gettextsettings function returns information about the current text settings used when text is displayed by the _grtext function. The information is stored in the textsettings structure indicated by the argument settings. The structure contains the following fields (all are short fields):
Fields | Meaning |
basevectorx | x-component of the current base vector |
basevectory | y-component of the current base vector |
path | current text path |
height | current text height (in pixels) |
width | current text width (in pixels) |
spacing | current text spacing (in pixels) |
horizalign | horizontal component of the current text alignment |
vertalign | vertical component of the current text alignment |
Returns : The _gettextsettings function returns information about the current graphics text settings.
See Also : _grtext, _setcharsize, _setcharspacing, _settextalign, _settextpath, _settextorient
Example :
#include <conio.h>
#include <graph.h>
main( )
{
struct textsettings ts;
_setvideomode( _VRES16COLOR );
_gettextsettings( &ts );
_grtext( 100, 100, "WATCOM" );
_setcharsize( 2 * ts.height, 2 * ts.width );
_grtext ( 100, 300, "Graphics" );
_setcharsize( ts.height, ts.width );
getch( );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
This manual describes the WATCOM C library for DOS, Windows, and OS/2, It includes the Standard C Library (as defined in the ANSI C Standard).
WATCOM C Language Reference manual describes the ANSI C Programming language and extensions to it which are supported by WATCOM C/C++ (32bit)