Watcom C Library : _pg_chart, _pg_chartms, _pg_chartpie, _pg_chartscatter, _pg_chartscatterms, _pg_defaultchart
Watcom C Reference/O - P - Q - R 2021. 8. 29. 17:58
Watcom C Library Reference : _pg_chart, _pg_chartms, _pg_chartpie, _pg_chartscatter, _pg_chartscatterms, _pg_defaultchart
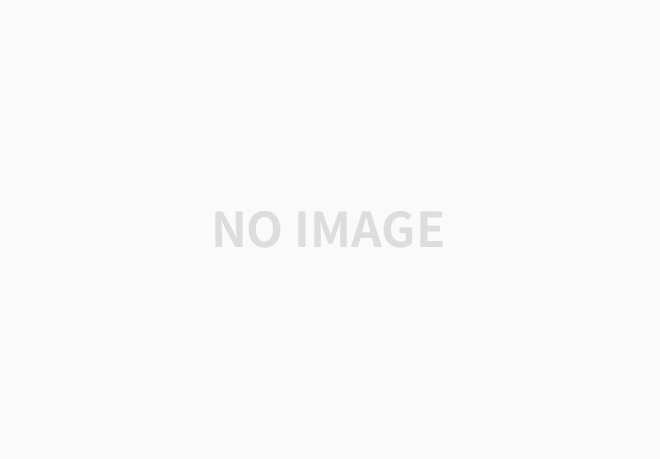
_pg_chart, _pg_chartms
Synopsis : #include <pgchart.h>
short _FAR _pg_chart( chartenv _FAR *env,
char _FAR *_FAR *cat,
float _FAR *values, short n );
short _FAR _pg_chartms( chartenv _FAR *env,
char _FAR *_FAR *cat,
float _FAR *values, short nseries,
short n, short dim,
char _FAR * _FAR *labels );
Description : The _pg_chart functions display either a single-series or a multi-series bar, column or line chart. The type of chart displayed and other chart options are contained in the env argument. The argument cat is an array of strings. These strings describe the categories against which the data in the values array is charted.
The _pg_chart function displays a bar, column or line chart from the single series of data contained in the values array. The argument n specifies the number of values to chart.
The _pg_chartms function displays a multi-series bar, column or line chart. The argument nseries specifies the number of series of data to chart. The argument values is assumed to be a two-dimensional array defined as follows:
float values[ nseries ][ dim ];
The number of values used from each series is given by the argument n, where n is less than or equal to dim. The argument labels is an array of strings. These strings describe each of the series and are used in the chart legend.
Returns : The _pg_chart functions return zero if successful; otherwise, a non-zero value is returned.
See Also : _pg_defaultchart, _pg_initchart, _pg_chartpie, _pg_chartscatter, _pg_analyzechart, _pg_analyzepie, _pg_analyzescatter
Example :
#include <graph.h>
#include <pgchart.h>
#include <string.h>
#include <conio.h>
#define NUM_VALUES 4
char _FAR *categories[NUM_VALUES] = { "Jan", "Feb", "Mar", "Apr" };
float values[NUM_VALUES] = { 20, 45, 30, 25 };
main( )
{
chartenv env;
_setvideomode( _VRES16COLOR );
_pg_initchart( );
_pg_defaultchart( ceny, _PG_COLUMNCHART, _PG_PLAINBARS );
strcpy( env.maintitle.title, "Column Chart" );
_pg_chart( &env, categories, values, NUM_VALUES );
getch( );
_setvideomode( _DEFAULTMODE );
}
produces the following :
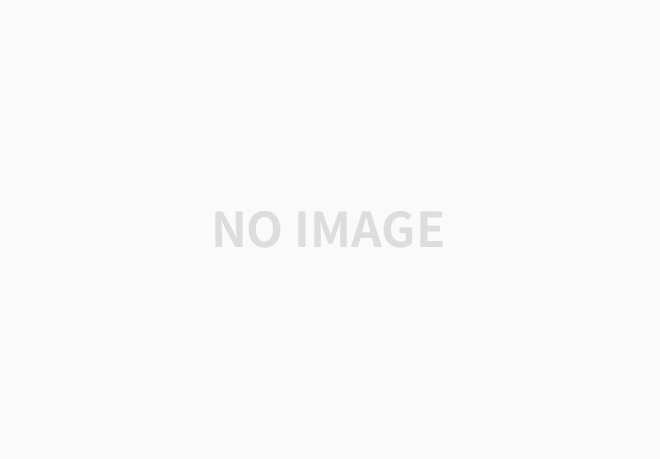
Classification : PC Graphics
Systems : _pg_chart - DOS, QNX
_pg_chartms - DOS, QNX
_pg_chartpie
Synopsis : #include <pgchart.h>
short _FAR _pg_chartpie( chartenv _FAR *env,
char _FAR * _FAR *cat,
float _FAR *values,
short _FAR *explode, short n );
Description : The _pg_chartpie function displays a pie chart. The chart is displayed using the options specified in the env argument.
The pie chart is created from the data contained in the values array. The argument n specifies the number of values to chart.
The argument cat is an array of strings. These strings describe each of the pie slices and are used in the chart legend. The argument explode is an array of values corresponding to each of the pie slices. For each non-zero element in the array, the corresponding pie slice is drawn "exploded", or slightly offset from the rest of the pie.
Returns : The _pg_chartpie function returns zero if successful; otherwise, a non-zero value is returned.
See Also : _pg_defaultchart, _pg_initchart, _pg_chart, _pg_chartscatter, _pg_analyzechart, _pg_analyzepie, _pg_analyzescatter
Example :
#include <graph.h>
#include <pgchart.h>
#include <string.h>
#include <conio.h>
#define NUM_VALUES 4
char _FAR *categories[NUM_VALUES] = { "Jan", "Feb", "Mar", "Apr" };
float values[NUM_VALUES] = { 20, 45, 30, 25 };
short explode[NUM_VALUES] = { 1, 0, 0, 0 };
main( )
{
chartenv env;
_setvideomode( _VRES16COLOR );
_pg_initchart( );
_pg_defaultchart ( &eny, _PG_PIECHART, _PG_NOPERCENT );
strcpy( eny.maintitle.title, "Pie Chart" );
_pg_chartpie ( &env, categories, values, explode, NUM_VALUES );
getch( );
_setvideomode( _DEFAULTMODE );
}
produces the following :
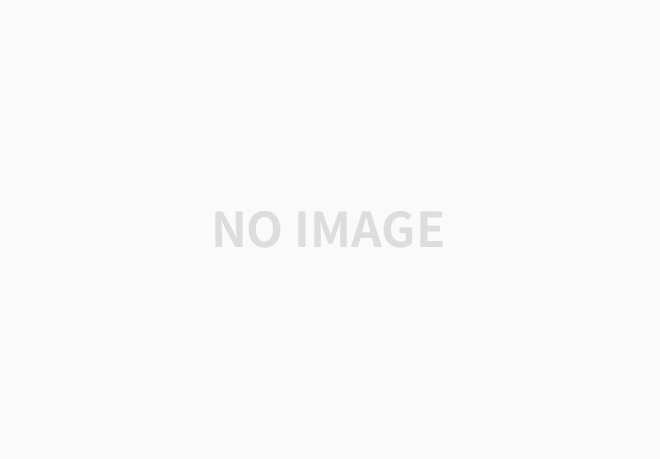
Classification : PC Graphics
Systems : DOS, QNX
_pg_chartscatter, _pg_chartscatterms
Synopsis : #include <pgchart.h>
short _FAR _pg_chartscatter( chartenv _FAR *env,
float _FAR *x, float _FAR *y, short n );
short _FAR _pg_chartscatterms( chartenv _FAR *env,
float _FAR *x, float _FAR *y,
short nseries, short n, short dim,
char _FAR * _FAR *labels );
Description : The _pg_chartscatter functions display either a single-series or a multi-series scatter chart. The chart is displayed using the options specified in the env argument.
The _pg_chartscatter function displays a scatter chart from the single series of data contained in the arrays x and y. The argument n specifies the number of values to chart.
The _pg_chartscatterms function displays a multi-series scatter chart. The argument nseries specifies the number of series of data to chart. The arguments x and y are assumed to be two-dimensional arrays defined as follows:
float x[ nseries ] [ dim ];
The number of values used from each series is given by the argument n, where n is less than or equal to dim. The argument labels is an array of strings. These strings describe each of the series and are used in the chart legend.
Returns : The _pg_chartscatter functions return zero if successful; otherwise, a non-zero value is returned.
See Also : _pg_defaultchart, _pg_initchart, _pg_chart, _pg_chartpie, _pg_analyzechart, _pg_analyzepie, _pg_analyzescatter
Example :
#include <graph.h>
#include <pgchart.h>
#include <string.h>
#include <conio.h>
#define NUM_VALUES 4
#define NUM_SERIES 2
char _FAR *labels[NUM_SERIES] = { "Jan", "Feb" };
float x[NUM_SERIES][NUM_VALUES] = { 5, 15, 30, 40, 10, 20, 30, 45 };
float y[NUM_SERIES][NUM_VALUES] = { 10, 15, 30, 45, 40, 30, 15, 5 };
main( )
{
chartenv env;
_setvideomode( _VRES16COLOR );
_pg_initchart( );
_pg_defaultchart( &eny, _PG_SCATTERCHART, _PG_POINTANDLINE );
strcpy( eny.maintitle.title, "Scatter Chart" );
_pg_chartscatterms( &env, x, y, NUM_SERIES, NUM_VALUES, NUM_VALUES, labels );
getch( );
_setvideomode( _DEFAULTMODE );
}
produces the following :
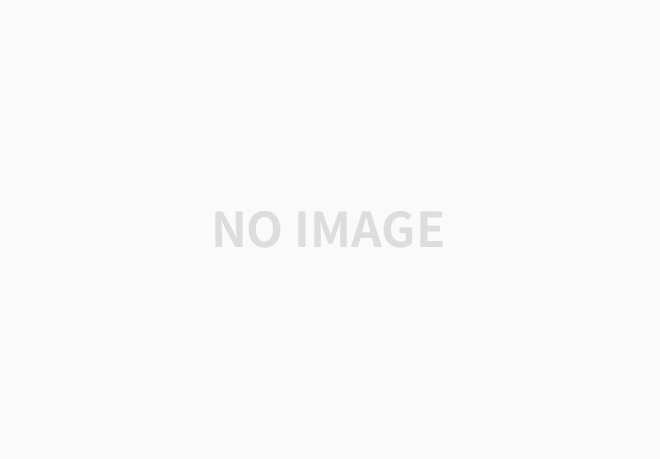
Classification : PC Graphics
Systems : _pg_chartscatter - DOS, QNX
_pg_chartscatterms - DOS, QNX
_pg_defaultchart
Synopsis : #include <pgchart.h>
short _FAR _pg_defaultchart( chartenv _FAR *env, short type, short style );
Description : The _pg_defaultchart function initializes the chart structure env to contain default values before a chart is drawn. All values in the chart structure are initialized, including blanking of all titles. The chart type in the structure is initialized to the value type, and the chart style is initialized to style.
The argument type can have one of the following values:
values | Meaning |
_PG_BARCHART | Bar chart (horizontal bars) |
_PG_COLUMNCHART | Column chart (vertical bars) |
_PG_LINECHART | Line chart |
_PG_SCATTERCHART | Scatter chart |
_PG_PIECHART | Pie chart |
Each type of chart can be drawn in one of two styles. For each chart type the argument style can have one of the following values:
Type | Style 1 | Style 2 |
Bar | _PG_PLAINBARS | _PG_STACKEDBARS |
Column | _PG_PLAINBARS | _PG_STACKEDBARS |
Line | _PG_POINTANDLINE | _PG_POINTONLY |
Scatter | _PG_POINTANDLINE | _PG_POINTONLY |
Pie | _PG_PERCENT | _PG_NOPERCENT |
For single-series bar and column charts, the chart style is ignored. The "plain" (clustered) and "stacked" styles only apply when there is more than one series of data. The "percent" style for pie charts causes percentages to be displayed beside each of the pie slices.
Returns : The _pg_defaultchart function returns zero if successful; otherwise, a non-zero value is returned.
See Also : _pg_initchart, _pg_chart, _pg_chartpie, _pg_chartscatter
Example :
#include <graph.h>
#include <pgchart.h>
#include <string.h>
#include <conio.h>
#define NUM_VALUES 4
char _FAR *categories[NUM_VALUES] = { "Jan", "Feb", "Mar", "Apr" };
float values[NUM_VALUES] = { 20, 45, 30, 25 };
main( )
{
chartenv env;
_setvideomode( _VRES16COLOR );
_pg_initchart( );
_pg_defaultchart ( &eny, _PG_COLUMNCHART, _PG_PLAINBARS );
strcpy( eny.maintitle.title, "Column Chart" );
_pg_chart( &env, categories, values, NUM_VALUES );
getch( );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
This manual describes the WATCOM C library for DOS, Windows, and OS/2, It includes the Standard C Library (as defined in the ANSI C Standard).
WATCOM C Language Reference manual describes the ANSI C Programming language and extensions to it which are supported by WATCOM C/C++ (32bit)