Watcom C Library : realloc Functions, _rectangle, _rectangle_w, _rectangle_wxy, _registerfonts, _remapallpalette, _remappalette
Watcom C Reference/O - P - Q - R 2021. 10. 4. 21:01
Watcom C Library Reference : realloc Functions, _rectangle, _rectangle_w, _rectangle_wxy, _registerfonts, _remapallpalette, _remappalette
realloc Functions
Synopsis : #include <stdlib.h> For ANSI compatibility (realloc only)
#include <malloc.h> Required for other function prototypes
void *realloc( void *old_blk, size_t size );
void __based(void) *_brealloc( __segment seg, void __based(void) *old_blk, size_t size );
void __far *_frealloc( void __far old_blk, size_t size );
void __near *_nrealloc( void __near *old_blk, size_t size );
Description : When the value of the old_blk argument is NULL, a new block of memory of size bytes is allocated.
If the value of size is zero, the corresponding free function is called to release the memory pointed to by old_blk.
Otherwise, the realloc function re-allocates space for an object of size bytes by either :
• shrinking the allocated size of the allocated memory block old_blk when size is sufficiently smaller than the size of old_blk.
• extending the allocated size of the allocated memory block old_blk if there is a large enough block of unallocated memory immediately following old_blk.
• allocating a new block and copying the contents of old_blk to the new block.
Because it is possible that a new block will be allocated, any pointers into the old memory should not be maintained. old_blk. These pointers will point to freed memory, with possible disastrous results, when a new block is allocated.
The function returns NULL when the memory pointed to by old_blk cannot be re-allocated. In this case, the memory pointed to by old_blk is not freed so care should be exercised to maintain a pointer to the old memory block.
buffer = (char *) realloc( buffer, 100 );
In the above example, buffer will be set to NULL if the function fails and will no longer point to the old memory block. If buffer was your only pointer to the memory block then you will have lost access to this memory.
Each function reallocates memory from a particular heap, as listed below:
Function | Heap |
realloc | Depends on data model of the program |
_brealloc | Based heap specified by seg value |
_frealloc | Far heap (outside the default data segment) |
_nrealloc | Near heap (inside the default data segment) |
In a small data memory model, the realloc function is equivalent to the _nrealloc function; in a large data memory model, the realloc function is equivalent to the _frealloc function.
Returns : The realloc functions return a pointer to the start of the re-allocated memory. The return value is NULL if there is insufficient memory available or if the value of the size argument is zero. The _brealloc function returns _NULLOFF if there is insufficient memory available or if the requested size is zero.
See Also : calloc Functions, _expand Functions, free Functions, halloc, hfree, malloc Functions, _msize Functions, sbrk
Example :
#include <stdlib.h>
#include <malloc.h>
void main( )
{
char *buffer;
char *new_buffer;
buffer = (char *)malloc( 80 );
new_buffer = (char *)realloc( buffer, 100 );
if( new_buffer == NULL ) {
/* not able to allocate larger buffer */
} else {
buffer = new_buffer;
}
}
Classification : realloc is ANSI; _brealloc, _frealloc, and _nrealloc are not ANSI
Systems : realloc - All
_brealloc - DOS/16, Win/16, QNX/16, OS/2 1.x(all)
_frealloc - DOS/16, Win/16, QNX/16, OS/2 1.x(all)
_nrealloc - DOS, Win, QNX, OS/2 1.x, OS/2 1.x(MT), OS/2 2.x, NT
_rectangle, _rectangle_w, _rectangle_wxy
Synopsis : #include <graph.h>
short _FAR _rectangle( short fill, short x1, short y1, short x2, short y2 );
short _FAR rectangle_w( short fill, double x1, double y1, double x2, double y2 );
short _FAR _rectangle_wxy( short fill, struct _wxycoord _FAR *p1, struct _wxycoord _FAR *p2 );
Description : The _rectangle functions draw rectangles. The _rectangle function uses the view coordinate system. The _rectangle_w and _rectangle_wxy functions use the window coordinate system.
The rectangle is defined with opposite corners established by the points (x1, y1) and (x2, y2).
The argument fill determines whether the rectangle is filled in or has only its outline drawn. The argument can have one of two values:
Values | Meaning |
_GFILLINTERIOR | fill the interior by writing pixels with the current plot action using the current color and the current fill mask |
_GBORDER | leave the interior unchanged; draw the outline of the figure with the current plot action using the current color and line style |
Returns : The _rectangle functions return a non-zero value when the rectangle was successfully drawn; otherwise, zero is returned.
See Also : _setcolor, _setfillmask, _setlinestyle, _setplotaction
Example :
#include <conio.h>
#include <graph.h>
main( )
{
_setvideomode( _VRES16COLOR );
_rectangle( _GBORDER, 100, 100, 540, 380 );
getch( );
_setvideomode( _DEFAULTMODE )
}
produces the following :
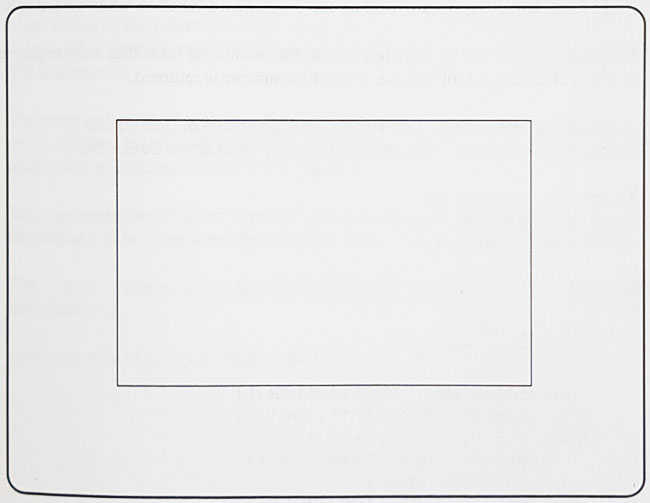
Classification : PC Graphics
Systems : rectangle - DOS, QNX
_rectangle_w - DOS, QNX
_rectangle_wxy - DOS, QNX
_registerfonts
Synopsis : #include <graph.h>
short _FAR _registerfonts( char _FAR *path );
Description : The _registerfonts function initializes the font graphics system. Fonts must be registered, and a font selected, before text can be displayed with the _outgtext function.
The argument path specifies the location of the font files. This argument is a file specification, and can contain drive and directory components and may contain wildcard characters. The _registerfonts function opens each of the font files specified and reads the font information. Memory is allocated to store the characteristics of the font. These font characteristics are used by the _setfont function when selecting a font.
Returns : The _registerfonts function returns the number of fonts that were registered if the function is successful; otherwise, a negative number is returned.
See Also : _unregisterfonts, _setfont, _getfontinfo, _outgtext, _getgtextextent, _setgtextvector, _getgtextvector
Example :
#include <conio.h>
#include <stdio.h>
#include <graph.h>
main( )
{
int i, n;
char buf[10];
_setvideomode( _VRES16COLOR );
n = _registerfonts ( "*. fon" );
for( i = 0; i < n; ++i ) {
sprintf( buf, "n%d", i );
_setfont ( buf );
moveto( 100, 100 );
_outgtext ( "WATCOM Graphics" );
getch( );
_clearscreen( _GCLEARSCREEN );
}
_unregisterfonts( );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
_remapallpalette
Synopsis : #include <graph.h>
short _FAR _remapallpalette( long _FAR *colors );
Description : The _remapallpalette function sets (or remaps) all of the colors in the palette. The color values in the palette are replaced by the array of color values given by the argument colors. This function is supported in all video modes, but only works with EGA, MCGA and VGA adapters.
The array colors must contain at least as many elements as there are supported colors. The newly mapped palette will cause the complete screen to change color wherever there is a pixel value of a changed color in the palette.
The representation of colors depends upon the hardware being used. The number of colors in the palette can be determined by using the _getvideoconfig function.
Returns : The _remapallpalette function returns (-1) if the palette is remapped successfully and zero otherwise.
See Also : _remappalette, _getvideoconfig
Example :
#include <conio.h>
#include <graph.h>
long colors[16] = {
_BRIGHTWHITE, _YELLOW, _LIGHTMAGENTA, _LIGHTRED,
_LIGHTCYAN, _LIGHTGREEN, _LIGHTBLUE, _GRAY, _WHITE,
_BROWN, _MAGENTA, _RED, _CYAN, _GREEN, _BLUE, BLACK,
};
main( )
{
int x, y;
_setvideomode( _VRES16COLOR );
for( y = 0; y < 4; ++y ) {
for ( x = 0; x < 4; ++x ) {
_setcolor ( x + 4 * y );
rectangle ( _GFILLINTERIOR, x * 160, y * 120,
( x + 1 ) * 160, ( y + 1 ) * 120 );
}
}
getch( );
_remapallpalette( &colors );
getch( );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
_remappalette
Synopsis : #include <graph.h>
long _FAR remappalette( short pixval, long color );
Description : The _remappalette function sets (or remaps) the palette color pixval to be the color color. This function is supported in all video modes, but only works with EGA, MCGA and VGA adapters.
The argument pixval is an index in the color palette of the current video mode. The argument color specifies the actual color displayed on the screen by pixels with pixel value pixval. Color values are selected by specifying the red, green and blue intensities that make up the color.
Each intensity can be in the range from 0 to 63, resulting in 262144 possible different colors. A given color value can be conveniently specified as a value of type long. The color value is of the form 0x00bbggrr, where bb is the blue intensity, gg is the green intensity and rr is the red intensity of the selected color. The file graph.h defines constants containing the color intensities of each of the 16 default colors.
The _remappalette function takes effect immediately. All pixels on the complete screen which have a pixel value equal to the value of pixval will now have the color indicated by the argument color.
Returns : The _remappalette function returns the previous color for the pixel value if the palette is remapped successfully; otherwise, (-1) is returned.
See Also : _remapallpalette, _setvideomode
Example :
#include <conio.h>
#include <graph.h>
long colors [16] = {
_BLACK, _BLUE, _GREEN, _CYAN, _RED, _MAGENTA,
_BROWN, _WHITE, _GRAY, _LIGHTBLUE, _LIGHTGREEN, _LIGHTCYAN,
_LIGHTRED, _LIGHTMAGENTA, _YELLOW, _BRIGHTWHITE
};
main( )
{
int col;
_setvideomode( _VRES16COLOR );
for ( col = 0; col < 16; ++col ) {
_remappalette( 0, colors[col] );
getch( );
}
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
This manual describes the WATCOM C library for DOS, Windows, and OS/2, It includes the Standard C Library (as defined in the ANSI C Standard).
WATCOM C Language Reference manual describes the ANSI C Programming language and extensions to it which are supported by WATCOM C/C++ (32bit)