Watcom C Library : _setpixel, _setpixel_w, _setplotaction, _settextalign, _settextcolor, _settextcursor
Watcom C Reference/S 2021. 10. 30. 19:53
Watcom C Library Reference : _setpixel, _setpixel_w, _setplotaction, _settextalign, _settextcolor, _settextcursor
_setpixel, _setpixel_w
Synopsis : #include <graph.h>
short _FAR _setpixel( short x, short y );
short _FAR _setpixel_w( double x, double y );
Description : The _setpixel function sets the pixel value of the point (x, y) using the current plotting action with the current color. The _setpixel function uses the view coordinate system. The _setpixel_w function uses the window coordinate system.
A pixel value is associated with each point. The values range from 0 to the number of colors (less one) that can be represented in the palette for the current video mode. The color displayed at the point is the color in the palette corresponding to the pixel number. For example, a pixel value of 3 causes the fourth color in the palette to be displayed at the point in question.
Returns : The _setpixel functions return the previous value of the indicated pixel if the pixel value can be set; otherwise, (-1) is returned.
See Also : _getpixel, _setcolor, _setplotaction
Example :
#include <conio.h>
#include <graph.h>
#include <stdlib.h>
void main( )
{
int x, y;
unsigned i;
_setvideomode( _VRES16COLOR );
_rectangle( _GBORDER, 100, 100, 540, 380 );
for( i = 0; i <= 60000; ++i ) {
x = 101 + rand( ) % 439;
y = 101 + rand( ) % 279;
_setcolor( _getpixel( x, y ) + 1 );
_setpixel( x, y );
}
getch( );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : _setpixel - DOS, QNX
_setpixel_w - DOS, QNX
_setplotaction
Synopsis : #include <graph.h>
short _FAR _setplotaction( short action );
Description : The _setplotaction function sets the current plotting action to the value of the action argument.
The drawing functions cause pixels to be set with a pixel value. By default, the value to be set is obtained by replacing the original pixel value with the supplied pixel value. Alternatively, the replaced value may be computed as a function of the original and the supplied pixel values.
The plotting action can have one of the following values:
Value | Meaning |
_GPSET | replace the original screen pixel value with the supplied pixel value |
_GAND | replace the original screen pixel value with the bitwise and of the original pixel value and the supplied pixel value |
_GOR | replace the original screen pixel value with the bitwise or of the original pixel value and the supplied pixel value |
_GXOR | replace the original screen pixel value with the bitwise exclusive-or of the original pixel value and the supplied pixel value. Performing this operation twice will restore the original screen contents, providing an efficient method to produce animated effects. |
Returns : The previous value of the plotting action is returned.
See Also : _getplotaction
Example :
#include <conio.h>
#include <graph.h>
void main( )
{
int old_act;
_setvideomode( _VRES16COLOR );
old_act = _getplotaction( );
_setplotaction( _GPSET );
_rectangle( _GFILLINTERIOR, 100, 100, 540, 380 );
getch( );
_setplotaction ( _GXOR );
_rectangle( _GFILLINTERIOR, 100, 100, 540, 380 );
getch();
_setplotaction( old_act );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
_settextalign
Synopsis : #include <graph.h>
void _FAR _settextalign( short horiz, short vert );
Description : The _settextalign function sets the current text alignment to the align function sets the current text alignment to the values specified by the arguments horiz and vert. When text is displayed with the _grtext function, it is aligned (justified) horizontally and vertically about the given point according to the current text alignment settings.
The horizontal component of the alignment can have one of the following values:
Value | Meaning |
_NORMAL | use the default horizontal alignment for the current setting of the text path |
_LEFT | the text string is left justified at the given point |
_CENTER | the text string is centred horizontally about the given point |
_RIGHT | the text string is right justified at the given point |
The vertical component of the alignment can have one of the following values:
Value | Meaning |
_NORMAL | use the default vertical alignment for the current setting of the text path |
_TOP | the top of the text string is aligned at the given point |
_CAP | the cap line of the text string is aligned at the given point |
_HALF | the text string is centred vertically about the given point |
_BASE | the base line of the text string is aligned at the given point |
_BOTTOM | the bottom of the text string is aligned at the given point |
The default is to use _LEFT alignment for the horizontal component unless the text path is _PATH_LEFT, in which case _RIGHT alignment is used. The default value for the vertical component is _TOP unless the text path is _PATH_UP, in which case _BOTTOM alignment is used.
Returns : The _settextalign function does not return a value.
See Also : _grtext, _gettextsettings
Example :
#include <conio.h>
#include <graph.h>
void main( )
{
_setvideomode( _VRES16COLOR );
_grtext( 200, 100, "WATCOM" );
_setpixel( 200, 100 );
_settextalign( _CENTER, _HALF );
_grtext( 200, 200, "Graphics" );
_setpixel( 200, 200 );
getch( );
_setvideomode( _DEFAULTMODE );
}
produces the following :
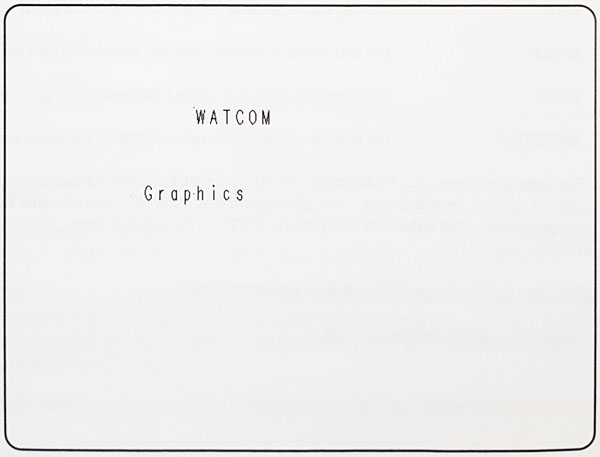
Classification : PC Graphics
Systems : DOS, QNX
_settextcolor
Synopsis : #include <graph.h>
short _FAR _settextcolor( short pixval );
Description : The _settextcolor function sets the current text color to be the color indicated by the pixel value of the pixval argument. This is the color value used for displaying text with the _outtext and _outmem functions. Use the _setcolor function to change the color of graphics output. The default text color value is set to 7 whenever a new video mode is selected.
The pixel value pixval is a number in the range 0-31. Colors in the range 0-15 are displayed normally. In text modes, blinking colors are specified by adding 16 to the normal color values. The following table specifies the default colors in color text modes.
Pixel Value | Color | Pixel Value | Color |
0 | Black | 8 | Gray |
1 | Blue | 9 | Light Blue |
2 | Green | 10 | Light Green |
3 | Cyan | 11 | Light Cyan |
4 | Red | 12 | Light Red |
5 | Magenta | 13 | Light Magenta |
6 | Brown | 14 | Yellow |
7 | White | 15 | Bright White |
Returns : The _settextcolor function returns the pixel value of the previous text color.
See Also : _gettextcolor, _outtext, _outmem, _setcolor
Example :
#include <conio.h>
#include <graph.h>
void main( )
{
int old_col;
long old_bk;
_setvideomode( _TEXTC80 );
old_col = _gettextcolor( );
old_bk = _getbkcolor( );
_settextcolor( 7 );
_setbkcolor( _BLUE );
_outtext( " WATCOM \nGraphics" );
_settextcolor( old_col );
_setbkcolor( old_bk );
getch( );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
_settextcursor
Synopsis : #include <graph.h>
short _FAR _settextcursor( short cursor );
Description : The _settextcursor function sets the attribute, or shape, of the cursor in text modes. The argument cursor specifies the new cursor shape. The cursor shape is selected by specifying the top and bottom rows in the character matrix. The high byte of cursor specifie the top row of the cursor; the low byte specifies the bottom row.
Some typical values for cursor are:
Cursor | Shape |
0x0607 | normal underline cursor |
0x0007 | full block cursor |
0x0407 | half-height block cursor |
0x2000 | no cursor |
Returns : The _settextcursor function returns the previous cursor shape when the shape is set successfully; otherwise, (-1) is returned.
See Also : _gettextcursor, -displaycursor
Example :
#include <conio.h>
#include <graph.h>
void main( )
{
int old_shape;
old_shape = _gettextcursor( );
_settextcursor( 0x0007 );
_outtext( "\nBlock cursor" );
getch( );
_settextcursor( 0x0407 );
_outtext( "\nHalf height cursor" );
getch( );
_settextcursor( 0x2000 );
_outtext( "\nNo cursor" );
getch( );
_settextcursor( old_shape );
}
Classification : PC Graphics
Systems : DOS, QNX
This manual describes the WATCOM C library for DOS, Windows, and OS/2, It includes the Standard C Library (as defined in the ANSI C Standard).
WATCOM C Language Reference manual describes the ANSI C Programming language and extensions to it which are supported by WATCOM C/C++ (32bit)