Watcom C Library : offsetof, onexit, open function
Watcom C Reference/O - P - Q - R 2021. 8. 17. 17:43
Watcom C Library Reference : offsetof, onexit, open
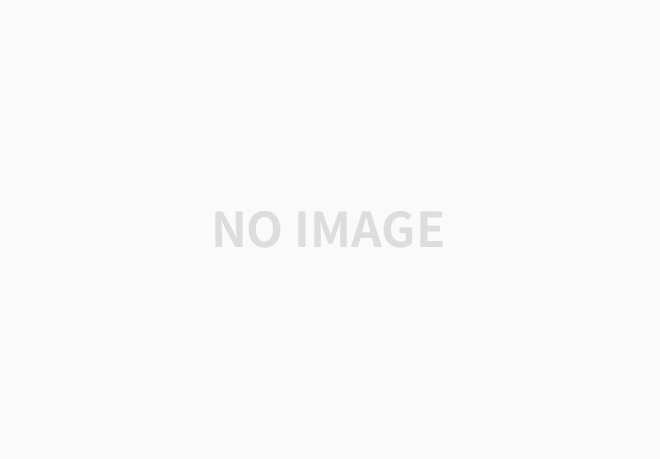
offsetof
Synopsis : #include <stddef.h>
size_t offsetof( composite, name );
Description : The offsetof macro returns the offset of the element name within the struct or union composite. This provides a portable method to determine the offset.
Returns : The offsetof function returns the offset of name.
Example :
#include <stdio.h>
#include <stddef.h>
struct new_def
{
char *first;
char second[10];
int third;
};
void main( )
{
printf( "first : %d second : %d third : %d\n",
offsetof( struct new_def, first ),
offsetof( struct new_def, second ),
offsetof( struct new_def, third ) );
}
produces the following :
In a small data model, the following would result:
first : 0 second : 2 third : 12
In a large data model, the following would result:
first : 0 second : 4 third : 14
Classification : ANSI
Systems : MACRO
onexit
Synopsis : #include <stdlib.h>
onexit_t onexit ( onexit_t func )
Description : The onexit function is passed the address of function func to be called when the program terminates normally. Successive calls to onexit create a list of functions that will be executed on a "last-in, first-out" basis. No more than 32 functions can be registered with the onexit function.
The functions have no parameters and do not return values.
NOTE: The onexit function is not an ANSI function. The ANSI standard function atexit does the same thing that onexit does and should be used instead of onexit where ANSI portability is concerned.
Returns : The onexit function returns func if the registration succeeds, NULL if it fails.
See Also : abort, atexit, exit, _exit
Example :
#include <stdio.h>
#include <stdlib.h>
void main( )
{
void func1(void), func2(void), func3(void);
onexit( func1 );
onexit( func2 );
onexit( func3 );
printf( "Do this first.\n" );
}
void func1( void ) { printf( "last.\n" ); }
void func2( void ) { printf( "this " ); }
void func3( void ) { printf( "Do " ); }
produces the following:
Do this first.
Do this last.
Classification : WATCOM
Systems : All
open
Synopsis : #include <sys\types.h>
#include <sys\stat.h>
#include <fcntl.h>
int open( const char *path, int access, ...);
Description : The open function opens a file at the operating system level. The name of the file to be opened is given by path. The file will be accessed according to the access mode specified by access. The optional argument is the file permissions to be used when the O_CREAT flag is on in the access mode.
The access mode is established by a combination of the bits defined in the <fcntl.h> header file. The following bits may be set:
Mode | Meaning |
O_RDONLY | permit the file to be only read. |
O_WRONLY | permit the file to be only written. |
O_RDWR | permit the file to be both read and written. |
O_APPEND | causes each record that is written to be written at the end of the file. |
O_CREAT | has no effect when the file indicated by filename already exists; otherwise, the file is created; |
O_TRUNC | causes the file to be truncated to contain no data when the file exists; has no effect when the file does not exist. |
O_BINARY | causes the file to be opened in binary mode which means that data will be transmitted to and from the file unchanged. |
O_TEXT | causes the file to be opened in text mode which means that carriage-return characters are written before any linefeed character that is written and causes carriage-return characters to be removed when encountered during reads. |
O_NOINHERIT | indicates that this file is not to be inherited by a child process. |
O_EXCL | indicates that this file is to be opened for exclusive access. If the file exists and O_CREAT was also specified then the open will fail (i.e., use O_EXCL to ensure that the file does not already exist). |
When neither O_TEXT nor O_BINARY are specified, the default value in the global variable _fmode is used to set the file translation mode. When the program begins execution, this variable has a value of O_TEXT.
O_CREAT must be specified when the file does not exist and it is to be written.
When the file is to be created (O_CREAT is specified), an additional argument must be passed which contains the file permissions to be used for the new file. The access permissions for the file or directory are specified as a combination of bits (defined in the <sys\stat.h> header file).
The following bits define permissions for the owner.
Permission | Meaning |
S_IRWXU | Read, write, execute/search |
S_IRUSR | Read permission |
S_IWUSR | Write permission |
S_IXUSR | Execute/search permission |
The following bits define permissions for the group.
Permission | Meaning |
S_IRWXG | Read, write, execute/search |
S_IRGRP | Read permission |
S_IWGRP | Write permission |
S_IXGRP | Execute/search permission |
The following bits define permissions for others.
Permission | Meaning |
S_IRWXO | Read, write, execute/search |
S_IROTH | Read permission |
S_IWOTH | Write permission |
S_IXOTH | Execute/search permission |
The following bits define miscellaneous permissions used by other implementations.
Permission | Meaning |
S_IREAD | is equivalent to S_IRUSR (read permission) |
S_IWRITE | is equivalent to S_IWUSR (write permission) |
S_IEXEC | is equivalent to S_IXUSR (execute/search permission) |
All files are readable with DOS; however, it is a good idea to set S_IREAD when read permission is intended for the file.
The open function applies the current file permission mask to the specified permissions (see umask).
Returns : If successful, open returns a handle for the file. When an error occurs while opening the file, -1 is returned.
Errors : When an error has occurred, errno contains a value indicating the type of error that has been detected.
Constant | Meaning |
EACCES | Access denied because path specifies a directory or a volume ID, or attempting to open a read-only file for writing |
EMFILE | No more handles available (too many open files) |
ENOENT | Path or file not found |
See Also : chsize, close, creat, dup, dup2, eof, exec Functions, filelength, fileno, fstat, isatty, lseek, read, setmode, sopen, stat, tell, write, umask
Example :
#include <sys\stat.h>
#include <sys\types.h>
#include <fcntl.h>
void main( )
{
int handle;
/* open a file for output */
/* replace existing file if it exists */
handle = open( "file", O_WRONLY | O_CREAT | O_TRUNC,
S_IRUSR | S_IWUSR | S_IRGRP | S_IWGRP );
/* read a file which is assumed to exist */
handle = open( "file", O_RDONLY );
/* append to the end of an existing file */
/* write a new file if file does not exist */
handle = open( "file", O_WRONLY | O_CREAT | O_APPEND,
S_IRUSR | S_IWUSR | S_IRGRP | S_IWGRP );
}
Classification : POSIX 1003.1
Systems : All
This manual describes the WATCOM C library for DOS, Windows, and OS/2, It includes the Standard C Library (as defined in the ANSI C Standard).
WATCOM C Language Reference manual describes the ANSI C Programming language and extensions to it which are supported by WATCOM C/C++ (32bit)