Watcom C Library Reference : sbrk, scanf
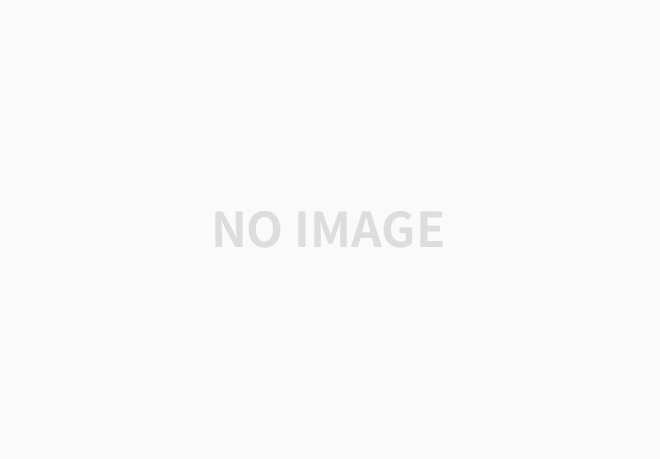
sbrk
Synopsis : #include <stdlib.h>
void *sbrk( int increment );
Description : The sbrk sets the "break" value for the program by adding the value of increment to the current break value. This increment may be positive or negative.
The "break" value is the address of the first byte of unallocated memory. When a program starts execution, the break value is placed following the code and constant data for the program. As memory is allocated, this pointer will advance when there is no freed block large enough to satisfy an allocation request.
A new process started with one of the spawn... or exec... functions is loaded following the break value. Consequently, decreasing the break value leaves more space available to the new process. Similarly, for a resident program (a program which remains in memory while another program executes), increasing the break value will leave more space available to be allocated by the resident program after other programs are loaded.
Returns : The function returns the old break value when the break value has been reset; otherwise. -1 is returned. When an error has occurred, errno contains a value indicating the type o that has been detected.
See Also : calloc Functions, _expand Functions, free Functions, halloc, hfree, malloc Functions, _msize Functions, realloc Functions
Example :
#include <stdio.h>
#include <stdlib.h>
void main( )
{
void *brk;
brk = sbrk( 0x0000 );
printf( "Old break value %p\n", brk );
brk = sbrk( -0x0100 );
printf( "Old break value %p\n", brk);
brk = sbrk( 0x0000 );
printf( "New break value %p\n", brk );
}
Classification : WATCOM
Systems : DOS, Win, QNX, OS/2 1.x, OS/2 1.x(MT), OS/2 2.x, NT
scanf
Synopsis : #include <stdio.h>
int scanf( const char *format, ... );
Description : The scanf function scans input from the file designated by stdin under control of the argument format. The format string is described below. Following the format string is the list of addresses of items to receive values.
Returns : The scanf function returns EOF when the scanning is terminated by reaching the end of the input stream. Otherwise, the number of input arguments for which values were successfully scanned and stored is returned.
See Also : cscanf, fscanf, sscanf, vcscanf, vfscanf, vscanf, vsscanf
Example :
To scan a date in the form "Saturday April 13 1999":
#include <stdio.h>
void main( )
{
int day, year;
char weekday[10], month[12];
scanf( "%s %s %d %d", weekday, month, &day, &year );
}
Format Control String : The format control string consists of zero or more format directives that specify
acceptable input file data. Subsequent arguments are pointers to various types of objects that are assigned values as the format string is processed.
A format directive can be a sequence of one or more white-space characters, an ordinary character, or a conversion specifier. An ordinary character in the format string is any character, other than a white-space character or the percent character(%), that is not part of a conversion specifier. A conversion specifier is a sequence of characters in the format string that begins with a percent character (%) and is followed, in sequence, by the following:
• an optional assignment suppression indicator: the asterisk character (*) | |
• an optional decimal integer that specifies the maximum field width to be scanned for the conversion | |
• an optional pointer-type specification: one of "N" or "F" | |
• an optional type length specification: one of "h", "l" or "L" | |
• a character that specifies the type of conversion to be performed: one of the characters cdefgionpsux [ |
As each format directive in the format string is processed, the directive may successfully complete, fail because of a lack of input data, or fail because of a matching error as defined by the particular directive. If end-of-file is encountered on the input data before any characters that match the current directive have been processed (other than leading white-space where permitted), the directive fails for lack of data. If end-of-file occurs after a matching character has been processed, the directive is completed (unless a matching error occurs), and the function returns without processing the next directive. If a directive fails because of an input character mismatch, the character is left unread in the input stream. Trailing white-space characters including new-line characters, are not read unless matched by a directive. When a format directive fails, or the end of the format string is encountered. the scanning is completed and the function returns.
When one or more white-space characters (space" ", horizontal tab "\t", vertical tab "\v", form feed "\f", carriage return "\r", new line or linefeed "\n") occur in the format string, input data up to the first non-white-space character is read, or until no more data remains. If no white-space characters are found in the input data, the scanning is complete and the function returns.
An ordinary character in the format string is expected to match the same character in the input stream.
A conversion specifier in the format string is processed as follows:
• for conversion types other than "[", "c" and "n", leading white-space characters are skipped | |
• for conversion types other than "n", all input characters, up to any specified maximum field length, that can be matched by the conversion type are read and converted to the appropriate type of value; the character immediately following the last character to be matched is left unread; if no characters are matched, the format directive fails | |
• unless the assignment suppression indicator ("*") was specified, the result of the conversion is assigned to the object pointed to by the next unused argument (if assignment suppression was specified, no argument is skipped); the arguments must correspond in number, type and order to the conversion specifiers in the format string |
A pointer-type specification is used to indicate the type of pointer used to locate the next argument to be scanned:
F : pointer is a far pointer
N : pointer is a near pointer
The pointer type defaults to that used for data in the memory model for which the program has been compiled.
A type length specifier affects the conversion as follows:
• "h" causes a "d", "i", "o", "u" or "x" (integer) conversion to assign the converted value to an object of type short int or unsigned short int | |
• "h" causes an "f" conversion to assign a fixed-point number to an object of type long consisting of a 16-bit integer part and a 16-bit fractional part. | |
• "h" causes an "n" (read length assignment) operation to assign the number of characters that have been read to an object of type unsigned short int | |
• "l" causes a "d", "i", "o", "u" or "x" (integer) conversion to assign the converted value to an object of type long int or unsigned long int | |
• "l" causes an "n" (read length assignment) operation to assign the number of characters that have been read to an object of type unsigned long int | |
• "l" causes an "e", "f" or "g" (floating-point) conversion to assign the converted value to an object of type double | |
• "L" causes an "e", "f" or "g" (floating-point) conversion to assign the converted value to an object of type long double |
The valid conversion type specifiers are:
c | Any sequence of characters in the input stream of the length specified by the field width, or a single character if no field width is specified, is matched. The argument is assumed to point to the first element of a character array of sufficient size to contain the sequence, without a terminating null character ('\0'). For a single character assignment, a pointer to a single object of type char is sufficient. |
d | A decimal integer, consisting of an optional sign, followed by one or more decimal digits, is matched. The argument is assumed to point to an object of type int. |
e, f, g | A floating-point number, consisting of an optional sign ("+" or "-"), followed by one or more decimal digits, optionally containing a decimal-point character, followed by an optional exponent of the form "e" or "E", an optional sign and one or more decimal digits, is matched. The exponent, if present, specifies the power of ten by which the decimal fraction is multiplied. The argument is assumed to point to an object of type float. |
i | An optional sign, followed by an octal, decimal or hexadecimal constant is matched. An octal constant consists of "0" and zero or more octal digits. A decimal constant consists of a non-zero decimal digit and zero or more decimal digits. A hexadecimal constant consists of the characters "0x" or "0X" followed by one or more (upper- or lowercase) hexadecimal digits. The argument is assumed to point to an object of type int. |
n | No input data is processed. Instead, the number of characters that have already been read is assigned to the object of type unsigned int that is pointed to by the argument. The number of items that have been scanned and assigned (the return value) is not affected by the "n" conversion type specifier. |
o | An octal integer, consisting of an optional sign, followed by one or more (zero or non-zero) octal digits, is matched. The argument is assumed to point to an object of type int. |
p | A hexadecimal integer, as described for "x" conversions below, is matched. The converted value is further converted to a value of type void* and then assigned to the object pointed to by the argument. |
s | A sequence of non-white-space characters is matched. The argument is assumed to point to the first element of a character array of sufficient size to contain the sequence and a terminating null character, which is added by the conversion operation. |
u | An unsigned decimal integer, consisting of one or more decimal digits, is matched. The argument is assumed to point to an object of type unsigned int. |
x | A hexadecimal integer, consisting of an optional sign, followed by an optional prefix "0x" or "0X", followed by one or more (upper- or lowercase) hexadecimal digits, is matched. The argument is assumed to point to an object of type int. |
[clc2...] | A sequence of characters, consisting of any of the characters c1, c2, ... called the scanset, in any order, is matched. c1 cannot be the caret character ('^'). If c1 is "]", that character is considered to be part of the scanset and a second "]" is required to end the format directive. The argument is assumed to point to the first element of a character array of sufficient size to contain the sequence and a terminating null character, which is added by the conversion operation. |
[^clc2...] | A sequence of characters, consisting of any of the characters other than the characters between the "^" and "]", is matched. As with the preceding conversion, if c1 is "]", it is considered to be part of the scanset and a second "]" ends the format directive. The argument is assumed to point to the first element of a character array of sufficient size to contain the sequence and a terminating null character, which is added by the conversion operation. |
A conversion type specifier of "%" is treated as a single ordinary character that matches a single "%" character in the input data. A conversion type specifier other than those listed above causes scanning to terminate the function to return.
The line
scanf( "%s %*f %3hx %d", name, &hexnum, &decnum )
with input
some_string 34.555e-3 abc1234
will copy "some_string" into the array name, skip 34.555e-3, assign 0xabc to hexnum and 1234 to decnum. The return value will be 3.
The program
#include <stdio.h>
void main( )
{
char string1[80], string2[80];
scanf( "%[ abcdefghijklmnopqrstuvwxyz"
"ABCDEFGHIJKLMNOPQRSTUVWZ ] %*2s%[^\n]", string1, string2 );
printf( "%s\n%s\n", string1, string2 )
}
with input
They may look alike, but they don't perform alike.
will assign
"They may look alike"
to string1, skip the comma (the "%*2s" will match only the comma; the following blank terminates that field), and assign
" but they don't perform alike."
to string2.
Classification : ANSI, (except for F and N modifiers)
Systems : All
This manual describes the WATCOM C library for DOS, Windows, and OS/2, It includes the Standard C Library (as defined in the ANSI C Standard).
WATCOM C Language Reference manual describes the ANSI C Programming language and extensions to it which are supported by WATCOM C/C++ (32bit)