Watcom C Library : umask, ungetc, ungetch, unlink, unlock, _unregisterfonts
Watcom C Reference/T ~ Z 2021. 11. 23. 17:47
Watcom C Library Reference : umask, ungetc, ungetch, unlink, unlock, _unregisterfonts
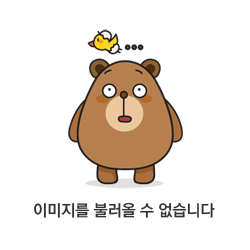
umask
Synopsis : #include <sys\types.h>
#include <sys\stat.h>
#include <fcntl.h>
#include <io.h>
int umask( int cmask );
Description : The umask function sets the process's file mode creation mask to cmask. The process's file mode creation mask is used during creat, open or sopen to turn off permission bits in the permission argument supplied. In other words, if a bit in the mask is on, then the corresponding bit in the file's requested permission value is disallowed.
The argument cmask is a constant expression involving the constants described below. The access permissions for the file or directory are specified as a combination of bits (defined in the <sys\stat.h> header file).
The following bits define permissions for the owner.
Permission | Meaning |
S_IRWXU | Read, write, execute/search |
S_IRUSR | Read permission |
S_IWUSR | Write permission |
S_IXUSR | Execute/search permission |
The following bits define permissions for the group.
Permission | Meaning |
S_IRWXG | Read, write, execute/search |
S_IRGRP | Read permission |
S_IWGRP | Write permission |
S_IXGRP | Execute/search permission |
The following bits define permissions for others.
Permission | Meaning |
S_IRWXO | Read, write, execute/search |
S_IROTH | Read permission |
S_IWOTH | Write permission |
S_IXOTH | Execute/search permission |
The following bits define miscellaneous permissions used by other implementations.
Permission | Meaning |
S_IREAD | is equivalent to S_IRUSR (read permission) |
S_IWRITE | is equivalent to S_IWUSR (write permission) |
S_IEXEC | is equivalent to S_IXUSR (execute/search permission) |
For example, if S_IRUSR is specified, then reading is not allowed (i.e., the file is write only). If S_IWUSR is specified, then writing is not allowed (i.e., the file is read only).
Returns : The umask function returns the previous value of cmask.
See Also : chmod, creat, mkdir, open, sopen
Example :
#include <sys\types.h>
#include <sys\stat.h>
#include <fontl.h>
#include <io.h>
void main( )
{
int old_mask;
/* set mask to create read-only files */
old_mask = umask( S_IWUSR | S_IWGRP I S_IWOTH | S_IXUSR | S_IXGRP | S_IXOTH );
}
Classification : POSIX 1003.1
Systems : All
ungetc
Synopsis : #include <stdio.h>
int ungetc( int c, FILE *fp );
Description : The ungetc function pushes the character specified by c back onto the input stream pointed to by fp. This character will be returned by the next read on the stream. The pushed-back character will be discarded if a call is made to the fflush function or to a file positioning function (fseek, fsetpos or rewind) before the next read operation is performed.
Only one character (the most recent one) of pushback is remembered.
The ungetc function clears the end-of-file indicator, unless the value of c is EOF.
Returns : The ungetc function returns the character pushed back.
See Also : fopen, getc
Example :
#include <stdio.h>
#include <ctype.h>
void main( )
{
FILE *fp;
int c;
long value;
fp = fopen( "file", "r" );
value = 0;
c = fgetc( fp );
while( isdigit( c ) ) {
value = value*10 + c - '0';
c = fgetc( fp );
}
ungetc( c, fp ); /* put last character back */
printf( "Value=%ld\n", value );
fclose( fp );
}
Classification : ANSI
Systems : All
ungetch
Synopsis : #include <conio.h>
int ungetch( int c );
Description : The ungetch function pushes the character specified by c back onto the input stream for the console. This character will be returned by the next read from the console (with getch or getche functions) and will be detected by the function kbhit. Only the last character returned in this way is remembered.
The ungetch function clears the end-of-file indicator, unless the value of c is EOF.
Returns : The ungetch function returns the character pushed back.
See Also : getch, getche, kbhit, putch
Example :
#include <stdio.h>
#include <ctype.h>
#include <conio.h>
void main( )
{
int c;
long value;
value = 0;
c = getche( );
while( isdigit( c ) ) {
value = value*10 + c - '0';
c = getche( );
}
ungetch( c );
printf( "Value=%ld\n", value );
}
Classification : WATCOM
Systems : All
unlink
Synopsis : #include <io.h>
int unlink( const char *path );
Description : The unlink function deletes the file whose name is the string pointed to by path. This function is equivalent to the remove function.
Returns : The unlink function returns zero if the operation succeeds, non-zero if it fails.
See Also : chdir, chmod, close, getcwd, mkdir, open, remove, rename, rmdir, stat
Example :
#include <io.h>
void main( )
{
unlink( "vm.tmp" );
}
Classification : POSIX 1003.1
Systems : All
unlock
Synopsis : #include <io.h>
int unlock( int handle, unsigned long offset, unsigned long nbytes );
Description : The unlock function unlocks nbytes amount of previously locked data in the file designated by handle starting at byte offset in the file. This allows other processes to lock this region of the file.
Multiple regions of a file can be locked, but no overlapping regions are allowed. You cannot unlock multiple regions in the same call, even if the regions are contiguous. All locked regions of a file should be unlocked before closing a file or exiting the program.
With DOS, locking is supported by version 3.0 or later. Note that SHARE.COM or SHARE.EXE must be installed.
Returns : The unlock function returns zero if successful, and -1 when an error occurs. When an error has occurred, errno contains a value indicating the type of error that has been detected.
See Also : lock, locking, open, sopen
Example :
#include <stdio.h>
#include <fcntl.h>
#include <io.h>
void main( )
{
int handle;
char buffer[20];
handle = open( "file", O_RDWR | O_TEXT );
if( handle != -1 ) {
if( lock( handle, 0L, 20L ) ) {
printf( "Lock failed\n" );
} else {
read( handle, buffer, 20 );
/* update the buffer here */
lseek( handle, 0L, SEEK_SET );
write( handle, buffer, 20 );
unlock( handle, 0L, 20L );
}
close( handle );
}
}
Classification : WATCOM
Systems : All
_unregisterfonts
Synopsis : #include <graph.h>
void _FAR _unregisterfonts( void );
Description : The _unregisterfonts function frees the memory previously allocated by the _registerfonts function. The currently selected font is also unloaded.
Attempting to use the _setfont function after calling _unregisterfonts will result in an error.
Returns : The _unregisterfonts function does not return a value.
See Also : _registerfonts, _setfont, _getfontinfo, _outgtext, _getgtextextent, _setgtextvector, _getgtextvector
Example :
#include <conio.h>
#include <stdio.h>
#include <graph.h>
void main( )
{
int i, n;
char buf[10];
_setvideomode( _VRES16COLOR );
n = _registerfonts( "*. fon" );
for( i = 0; i < n; ++i ) {
sprintf( buf, "n%d", i );
_setfont( buf );
_moveto( 100, 100 );
_outgtext( "WATCOM Graphics" );
getch( );
_clearscreen( _GCLEARSCREEN );
}
_unregisterfonts( );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
This manual describes the WATCOM C library for DOS, Windows, and OS/2, It includes the Standard C Library (as defined in the ANSI C Standard).
WATCOM C Language Reference manual describes the ANSI C Programming language and extensions to it which are supported by WATCOM C/C++ (32bit)
'Watcom C Reference > T ~ Z' 카테고리의 다른 글
Watcom C Library : wait, wcstombs, wctomb, _wrapon, write (0) | 2021.12.01 |
---|---|
Watcom C Library : vfscanf, vprintf, vscanf, vsprintf, vsscanf (0) | 2021.11.29 |
Watcom C Library : _vbprintf, vcprintf, vcscanf, vfprintf (0) | 2021.11.28 |
Watcom C Library : utime, utoa, va_arg, va_end, va_start (0) | 2021.11.26 |
Watcom C Library : tmpnam, tolower, toupper, tzset, ultoa (0) | 2021.11.22 |
Watcom C Library : tan, tanh, tell, time, tmpfile (0) | 2021.11.20 |