Watcom C Library : _settextorient, _settextpath, _settextposition, _settextrows, _settextwindow, setvbuf
Watcom C Reference/S 2021. 11. 2. 18:16
Watcom C Library Reference : _settextorient, _settextpath, _settextposition, _settextrows, _settextwindow, setvbuf
_settextorient
Synopsis : #include <graph.h>
void _FAR _settextorient( short vecx, short vecy );
Description : The _settextorient function sets the current text orientation to the vector specified by the arguments (vecx, vecy). The text orientation specifies the direction of the base-line vector when a text string is displayed with the _grtext function. The default text orientation, for normal left-to-right text, is the vector (1, 0).
Returns : The _settextorient function does not return a value.
See Also : _grtext, _gettextsettings
Example :
#include <conio.h>
#include <graph.h>
void main( )
{
_setvideomode( _VRES16COLOR );
_grtext( 200, 100, "WATCOM" );
_settextorient( 1, 1 );
_grtext( 200, 200, "Graphics" );
getch( );
_setvideomode( _DEFAULTMODE );
}
produces the following :
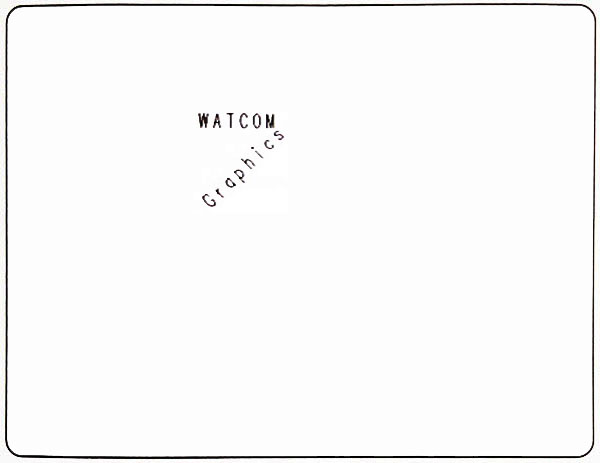
Classification : PC Graphics
Systems : DOS, QNX
_settextpath
Synopsis : #include <graph.h>
void _FAR _settextpath( short path );
Description : The _settextpath function sets the current text path to have the value of the path argument. The text path specifies the writing direction of the text displayed by the _grtext function. The argument can have one of the following values:
Value | Meaning |
_PATH_RIGHT | subsequent characters are drawn to the right of the previous character |
_PATH_LEFT | subsequent characters are drawn to the left of the previous character |
_PATH_UP | subsequent characters are drawn above the previous character |
_PATH_DOWN | subsequent characters are drawn below the previous character |
The default value of the text path is _PATH_RIGHT.
Returns : The _settextpath function does not return a value.
See Also : _grtext, _gettextsettings
Example :
#include <conio.h>
#include <graph.h>
void main( )
{
_setvideomode( _VRES16COLOR );
_grtext( 200, 100, "WATCOM" );
_settextpath( _PATH_DOWN );
_grtext( 200, 200, "Graphics" );
getch( );
_setvideomode( _DEFAULTMODE );
}
produces the following :

Classification : PC Graphics
Systems : DOS, QNX
_settextposition
Synopsis : #include <graph.h>
struct rccoord _FAR _settextposition( short row, short col ) ;
Description : The _settextposition function sets the current output position for text to be (row, col) where this position is in terms of characters, not pixels.
The text position is relative to the current text window. It defaults to the top left corner of the screen, (1, 1), when a new video mode is selected, or when a new text window is set. The position is updated as text is drawn with the _outtext and _outmem functions.
Note that the output position for graphics output differs from that for text output. The output position for graphics output can be set by use of the _moveto function.
Returns : The _settextposition function returns, as an rccoord structure, the previous output position for text.
See Also : _gettextposition, _outtext, _outmem, _settextwindow, _moveto
Example :
#include <conio.h>
#include <graph.h>
void main( )
{
struct rccoord old_pos;
_setvideomode( _TEXTC80 );
old_pos = _gettextposition( );
_settextposition( 10, 40 );
_outtext( "WATCOM Graphics" );
_settextposition( old_pos.row, old_pos.col );
getch( );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
_settextrows
Synopsis : #include <graph.h>
short _FAR _settextrows( short rows );
Description : The _settextrows function selects the number of rows of text displayed on the screen. The number of rows is specified by the argument rows. Computers equipped with EGA, MCGA and VGA adapters can support different numbers of text rows. The number of rows that can be selected depends on the current video mode and the type of monitor attached.
If the argument rows has the value _MAXTEXTROWS, the maximum number of text rows will be selected for the current video mode and hardware configuration. In text modes the maximum number of rows is 43 for EGA adapters, and 50 for MCGA and VGA adapters. Some graphics modes will support 43 rows for EGA adapters and 60 rows for MCGA and VGA adapters.
Returns : The _settextrows function returns the number of screen rows when the number of rows is set successfully; otherwise, zero is returned.
See Also : _getvideoconfig, _setvideomode, _setvideomoderows
Example :
#include <conio.h>
#include <graph.h>
#include <stdio.h>
int valid_rows[] = { 14, 25, 28, 30, 34, 43, 50, 60 };
void main( )
{
int i, j, rows;
char buf[80];
for( i = 0; i < 8; ++i ) {
rows = valid_rows[i];
if( _settextrows( rows ) == rows ) {
for ( j = 1; j <= rows; ++j ) ) {
sprintf( buf, "Line %d", j );
_settextposition( j, 1 );
_outtext( buf );
}
getch( );
}
}
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
_settextwindow
Synopsis : #include <graph.h>
void _FAR _settextwindow( short row1, short col1, short row2, short col2 );
Description : The _settextwindow function sets the text window to be the rectangle with a top left corner at (row1, col1) and a bottom right corner at (row2, col2). These coordinates are in terms of characters not pixels.
The initial text output position is (1, 1). Subsequent text positions are reported (by the _gettextposition function) and set (by the _outtext, _outmem and _settextposition functions) relative to this rectangle.
Text is displayed from the current output position for text proceeding along the current row and then downwards. When the window is full, the lines scroll upwards one line and then text is displayed on the last line of the window.
Returns : The _settextwindow function does not return a value.
See Also : _gettextposition, _outtext, _outmem, _settextposition
Example :
#include <conio.h>
#include <graph.h>
#include <stdio.h>
void main( )
{
int i;
short r1, c1, r2, c2;
char buf[80];
_setvideomode( _TEXTC80 );
_gettextwindow( &r1, &c1, &r2, &c2 );
_settextwindow( 5, 20, 20, 40 );
for ( i = 1; i <= 20; ++i ) {
sprintf( buf, "Line %d\n", i);
_outtext( buf );
}
getch( );
_settextwindow( r1, c1, r2, c2 );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
setvbuf
Synopsis : #include <stdio.h>
int setvbuf( FILE *fp, char *buf, int mode, size_t size );
Description : The setvbuf function can be used to associate a buffer with the file designated by fp. If this function is used, it must be called after the file has been opened and before it has been read or written. The argument mode determines how the file fp will be buffered, as follows:
Mode | Meaning |
_IOFBF | causes input/output to be fully buffered. |
_IOLBF | causes output to be line buffered (the buffer will be flushed when a new-line character is written, when the buffer is full, or when input is requested. |
_IONBF | causes input/output to be completely unbuffered. |
If the argument buf is not NULL, the array to which it points will be used instead of an automatically allocated buffer. The argument size specifies the size of the array.
Returns : The setvbuf function returns zero on success, or a non-zero value if an invalid value is given for mode or size.
See Also : fopen, setbuf
Example :
#include <stdio.h>
#include <stdlib.h>
void main( )
{
char *buf;
FILE *fp;
fp = fopen( "file", "r" );
buf = malloc( 1024 );
setvbuf( fp, buf, _IOFBF, 1024 );
}
Classification : ANSI
Systems : All
This manual describes the WATCOM C library for DOS, Windows, and OS/2, It includes the Standard C Library (as defined in the ANSI C Standard).
WATCOM C Language Reference manual describes the ANSI C Programming language and extensions to it which are supported by WATCOM C/C++ (32bit)