Watcom C Library : _setbkcolor, setbuf, _setcharsize, _setcharsize_w, _setcharspacing, _setcharspacing_w, _setcliprgn
Watcom C Reference/S 2021. 10. 22. 19:45
Watcom C Library Reference : _setbkcolor, setbuf, _setcharsize, _setcharsize_w, _setcharspacing, _setcharspacing_w, _setcliprgn
_setbkcolor
Synopsis : #include <graph.h>
long _FAR _setbkcolor( long color );
Description : The _setbkcolor function sets the current background color to be that of the color argument. In text modes, the background color controls the area behind each individual character. In graphics modes, the background refers to the entire screen. The default background color is 0.
When the current video mode is a graphics mode, any pixels with a zero pixel value will change to the color of the color argument. When the current video mode is a text mode, nothing will immediately change; only subsequent output is affected.
Returns : The _setbkcolor function returns the previous background color.
See Also : _getbkcolor
Example :
#include <conio.h>
#include <graph.h>
long colors [16] = {
_BLACK, _BLUE, _GREEN, _CYAN,
_RED, _MAGENTA, _BROWN, _WHITE,
_GRAY, _LIGHTBLUE, _LIGHTGREEN, _LIGHTCYAN,
_LIGHTRED, _LIGHTMAGENTA, _YELLOW, _BRIGHTWHITE
};
void main( )
{
long old_bk;
int bk;
_setvideomode( _VRES16COLOR );
old_bk = _getbkcolor( );
for( bk = 0; bk < 16; ++bk ) {
_setbkcolor( colors [bk] );
getch( );
}
_setbkcolor( old_bk );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
setbuf
Synopsis : #include <stdio.h>
void setbuf( FILE *fp, char *buffer );
Description : The setbuf function can be used to associate a buffer with the file designated by fp. If this function is used, it must be called after the file has been opened and before it has been read or written. If the argument buffer is NULL, then all input/output for the file fp will be completely unbuffered. If the argument buffer is not NULL, then it must point to an array that is at least BUFSIZ characters in length, and all input/output will be fully buffered.
Returns : The setbuf function returns no value.
See Also : fopen, setvbuf
Example :
#include <stdio.h>
#include <stdlib.h>
void main( )
{
char *buffer;
FILE *fp;
fp = fopen( "file", "r" );
buffer = (char *) malloc( BUFSIZ );
setbuf( fp, buffer );
/* . */
/* . */
/* . */
fclose( fp );
}
Classification : ANSI
Systems : All
_setcharsize, _setcharsize_w
Synopsis : #include <graph.h>
void _FAR _setcharsize( short height, short width );
void _FAR _setcharsize_w( double height, double width );
Description : The _setcharsize functions set the character height and width to the values specified by the arguments height and width. For the _setcharsize function, the arguments height and width represent a number of pixels. For the _setcharsize_w function, the arguments height and width represent lengths along the y-axis and x-axis in the window coordinate system.
These sizes are used when displaying text with the _grtext function. The default character sizes are dependent on the graphics mode selected, and can be determined by the _gettextsettings function.
Returns : The _setcharsize functions do not return a value.
See Also : _grtext, _gettextsettings
Example :
#include <conio.h>
#include <graph.h>
void main( )
{
struct textsettings ts;
_setvideomode( _VRES16COLOR );
_gettextsettings( &ts );
_grtext( 100, 100, "WATCOM" );
_setcharsize( 2 * ts.height, 2 * ts.width );
_grtext( 100, 300, "Graphics" );
_setcharsize( ts.height, ts.width );
getch( );
_setvideomode( DEFAULTMODE );
}
produces the following :
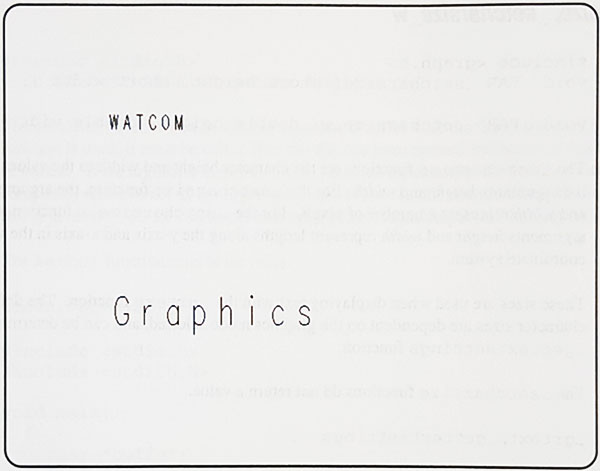
Classification : PC Graphics
Systems : _setcharsize - DOS, QNX
_setcharsize_w - DOS, QNX
_setcharspacing, _setcharspacing_w
Synopsis : #include <graph.h>
void _FAR setcharspacing( short space );
void _FAR _setcharspacing_w( double space );
Description : The _setcharspacing functions set the current character spacing to have the value of the argument space. For the _setcharspacing function, space represents a number of pixels. For the _setcharspacing_w function, space represents a length along the x-axis in the window coordinate system.
The character spacing specifies the additional space to leave between characters when a text string is displayed with the _grtext function. A negative value can be specified to cause the characters to be drawn closer together. The default value of the character spacing is 0.
Returns : The _setcharspacing functions do not return a value.
See Also : _grtext, _gettextsettings
Example :
#include <conio.h>
#include <graph.h>
void main( )
{
_setvideomode( _VRES16COLOR );
_grtext( 100, 100, "WATCOM" );
_setcharspacing( 20 );
_grtext( 100, 300, "Graphics" );
getch( );
_setvideomode( _DEFAULTMODE );
}
produces the following :
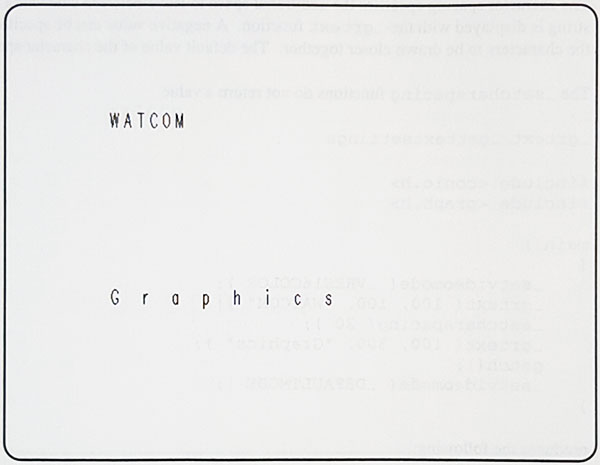
Classification : PC Graphics
Systems : _setcharspacing - DOS, QNX
_setcharspacing_w - DOS, QNX
_setcliprgn
Synopsis : #include <graph.h>
void _FAR _setcliprgn( short x1, short y1, short x2, short y2 );
Description : The _setcliprgn function restricts the display of graphics output to the clipping region.
This region is a rectangle whose opposite corners are established by the physical points (x1, y1) and (x2, y2).
The _setcliprgn function does not affect text output using the _outtext and _outmem functions. To control the location of text output, see the _settextwindow function.
Returns : The _setcliprgn function does not return a value.
See Also : _settextwindow, _setvieworg, _setviewport
Example :
#include <conio.h>
#include <graph.h>
void main( )
{
short x1, y1, x2, y2;
_setvideomode( _VRES16COLOR );
_getcliprgn( &x1, &y1, &x2, &y2 );
_setcliprgn( 130, 100, 510, 380 );
_ellipse( _GBORDER, 120, 90, 520, 390 );
getch( );
_setcliprgn( x1, y1, x2, y2 );
_setvideomode( _DEFAULTMODE );
}
Classification : PC Graphics
Systems : DOS, QNX
This manual describes the WATCOM C library for DOS, Windows, and OS/2, It includes the Standard C Library (as defined in the ANSI C Standard).
WATCOM C Language Reference manual describes the ANSI C Programming language and extensions to it which are supported by WATCOM C/C++ (32bit)